Objective C and Foundation
Language and Programming Basics
Moving from Language to Application
Workspace Areas - The Toolbar and Tab Bar
Using Frameworks and Libraries
Functions and Data Structures
Using the Debugger
Loops
Creating your First Object
Understanding how Objects Behave
Methods and Messages
Objects and Memory
NSString
NSArray
Accessor Methods
Properties - Defining and Working with Property Lists
Inheritance Hierarchy
Object Instance - Variables and Properties
Class Extensions
Bitwise Operations
How Operators work in Objective-C
Accessing Data with Pointers
Preventing Memory Leaks
Collection Classes
Constants - Preprocessor Directives
Callbacks
Using Blocks
Protocols
Moving from Functions and Global Data to Objects and Classes
Replacing Numbers with Objects
Encapsulating Objects
Memory Management
Adding a Plist to your Application
Creating a Mutable Dictionary
A basic Init Method
Multiple Initializers
More on Property Attributes
Key Value Coding - Non Object Types
Key Value Observing - Using Context
Triggering Notifications
C Strings - Converting to and fro NSString
Running from the Command Line
Creating Applications
Event Driven Applications - your First iOS Application
Cocoa Applications
Creating the Interface
The Implementation - Coding the Methods
Exploring the Program Logic
Understanding Naming Conventions
Using ID and NILL
Adding an iPhone User Interface
Introduction To Swift
Swift Development Environment
Swift’s Fundamental Data Types
Overview of:
Using Operators
Collection Types
Optional Values
Program Flow with Conditionals
Iterating Code with Loops
Performing Actions
Structure and Classes
The Power of Enums
Customizing Classes, Structures and Enums
Properties
Extensions
Optional Chaining
Adding Interoperability with Objective-C
User Interfaces
Standard Library Functions
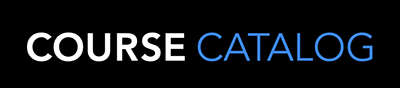
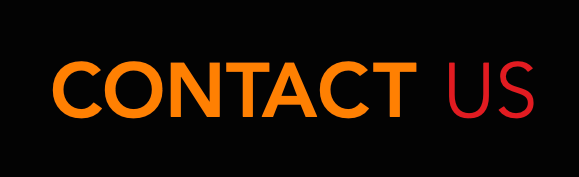